Shallow copy (also know as Address Copy):
Shallow copies duplicate as little as possible. A shallow copy of a collection is a copy of the collection structure, not the elements. With a shallow copy, two collections now share the individual elements.
Deep copy:
Deep copies duplicate everything. A deep copy of a collection is two collections with all of the elements in the original collection duplicated.
If
B and A point to the same memory location
If
B and A point to different memory locations
B memory address is same as A's
B has same contents as A's

For a true deep copy (Array of Arrays) you will need
Shallow copy is also known as address copy. In this process you only copy address not actual data while in deep copy you copy data.
Suppose there are two objects A and B. A is pointing to a different array while B is pointing to different array. Now what I will do is following to do shallow copy.
Char *A = {‘a’,’b’,’c’};
Char *B = {‘x’,’y’,’z’};
B = A;
Now B is pointing is at same location where A pointer is pointing.Both A and B in this case sharing same data. if change is made both will get altered value of data.Advantage is that coping process is very fast and is independent of size of array.
while in deep copy data is also copied. This process is slow but Both A and B have their own copies and changes made to any copy, other will copy will not be affected.
[Ref: https://iphonecodecenter.wordpress.com/2013/08/26/difference-between-shallow-copy-and-deep-copy/ ]
References:
Shallow copies duplicate as little as possible. A shallow copy of a collection is a copy of the collection structure, not the elements. With a shallow copy, two collections now share the individual elements.
Deep copy:
Deep copies duplicate everything. A deep copy of a collection is two collections with all of the elements in the original collection duplicated.
If
B
is a shallow copy of A
, then it is like B = [A assign];
B and A point to the same memory location
If
B
is a deep copy of A
, then it is like B = [A copy];
B and A point to different memory locations
B memory address is same as A's
B has same contents as A's

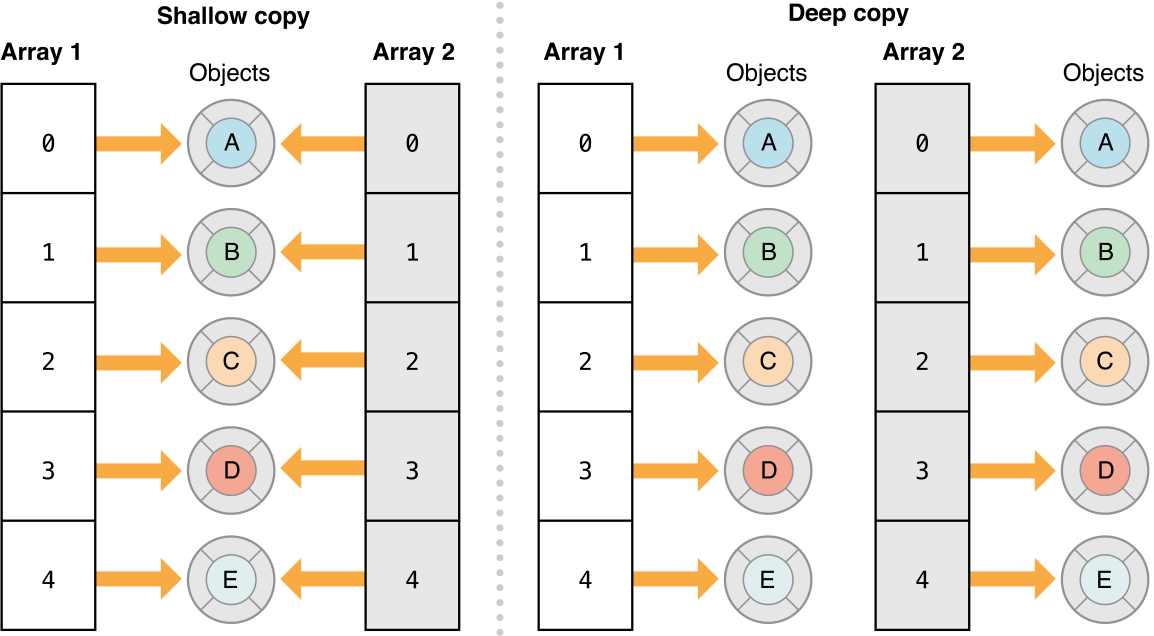
Copy by default gives a shallow copy
That is because callingcopy
is the same as copyWithZone:NULL
also known as copying with the default zone. The copy
call does not result in a deep copy. In most cases it would give you a
shallow copy, but in any case it depends on the class. For a thorough
discussion I recommend the Collections Programming Topics on the Apple Developer site. initWithArray:CopyItems: gives a one-level deep copy
NSArray *deepCopyArray = [[NSArray alloc] initWithArray:someArray copyItems:YES];
NSCoding
is the Apple recommended way to provide a deep copy
For a true deep copy (Array of Arrays) you will need NSCoding
and archive/unarchive the object:NSArray *trueDeepCopyArray = [NSKeyedUnarchiver unarchiveObjectWithData:[NSKeyedArchiver archivedDataWithRootObject:oldArray]];
Other way of explanation
Shallow copy is also known as address copy. In this process you only copy address not actual data while in deep copy you copy data.
Suppose there are two objects A and B. A is pointing to a different array while B is pointing to different array. Now what I will do is following to do shallow copy.
Char *A = {‘a’,’b’,’c’};
Char *B = {‘x’,’y’,’z’};
B = A;
Now B is pointing is at same location where A pointer is pointing.Both A and B in this case sharing same data. if change is made both will get altered value of data.Advantage is that coping process is very fast and is independent of size of array.
while in deep copy data is also copied. This process is slow but Both A and B have their own copies and changes made to any copy, other will copy will not be affected.
[Ref: https://iphonecodecenter.wordpress.com/2013/08/26/difference-between-shallow-copy-and-deep-copy/ ]
References:
No comments:
Post a Comment